CSS Sprites: The Hidden Performance Gem Behind Facebook's Icons
Have you ever wondered how major websites like Facebook manage to load dozens of icons instantly without any delay? The secret lies in a clever technique called CSS Sprites. In this article, we'll dive deep into what CSS Sprites are, how they work, and why they remain relevant even in today's modern web landscape.
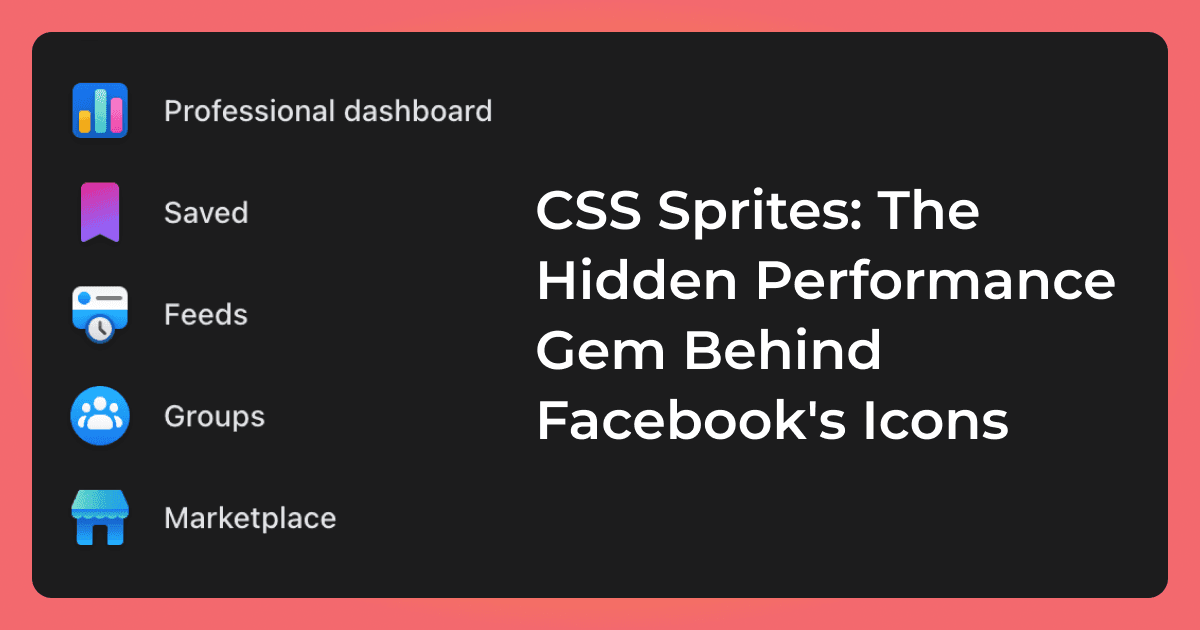
What Are CSS Sprites?
CSS Sprites is a technique where multiple images or icons are combined into a single, larger image file, but only specific portions are displayed at a time. Think of it like a film strip, where each frame contains a different image, but you only see one frame through the projector's window at any moment.
How Facebook Implements CSS Sprites
Let's look at a real example from Facebook's implementation:
.menu-icon {
background-image: url(https://static.xx.fbcdn.net/rsrc.php/v4/y-/r/cJdNYgqUyfE.png);
background-position: 0 -409px;
background-size: 37px 592px;
width: 36px;
height: 36px;
background-repeat: no-repeat;
display: inline-block;
}
As you can see, Facebook uses a single image file (cJdNYgqUyfE.png
) for multiple icons. https://static.xx.fbcdn.net/rsrc.php/v4/y-/r/cJdNYgqUyfE.png
This CSS does several crucial things:
- It loads a single image file containing multiple icons
- The background-size indicates this is a tall image (592px height) containing multiple icons stacked vertically
- By adjusting background-position, different icons can be displayed within the 36x36 pixel "window"
To show different icons, Facebook simply changes the vertical position. For example:
.home-icon {
background-position: 0 0;
} /* Shows the home icon */
.messages-icon {
background-position: 0 -36px;
} /* Shows the messages icon */
.groups-icon {
background-position: 0 -72px;
} /* Shows the groups icon */
The Benefits of CSS Sprites
1. Dramatic Performance Improvements
The most significant advantage is performance. Consider a navigation menu with 10 icons:
Traditional approach:
- 10 separate HTTP requests
- 10 separate round trips to the server
- 10 separate files to cache
CSS Sprites approach:
- 1 HTTP request
- 1 round trip to the server
- 1 file to cache
For high-traffic sites like Facebook, this reduction in HTTP requests translates to enormous savings in server resources and improved user experience.
2. Smoother User Experience
When loading individual icons, users might experience icons popping in at different times as each file loads. With sprites, all icons are available immediately once the sprite sheet loads, creating a smoother, more professional appearance.
3. Efficient Browser Caching
Browsers can more efficiently cache one larger file than many smaller files. This means return visitors to your site will experience even faster load times, as the browser only needs to check one cache entry instead of many.
Creating Your Own Sprite Sheet
Here's a practical example of creating and implementing a basic sprite sheet:
- First, create your sprite sheet by stacking icons vertically:
/* sprite-sheet.css */
.icon {
background-image: url('sprite-sheet.png');
background-repeat: no-repeat;
width: 32px;
height: 32px;
display: inline-block;
}
.icon-home {
background-position: 0 0;
}
.icon-search {
background-position: 0 -32px;
}
.icon-settings {
background-position: 0 -64px;
}
- Use it in your HTML:
<div class="icon icon-home"></div>
<div class="icon icon-search"></div>
<div class="icon icon-settings"></div>
Modern Considerations
While HTTP/2 and HTTP/3 have reduced the performance impact of multiple requests, CSS Sprites remain valuable for several reasons:
- Reduced Server Load: Even with multiplexing, processing fewer requests reduces server load.
- Bandwidth Efficiency: One larger file often compresses better than many small files.
- Legacy Support: Many users still access sites through HTTP/1.1.
- Mobile Optimization: Fewer requests mean less battery drain on mobile devices.
Tools and Best Practices
To implement CSS Sprites effectively:
- Use sprite generation tools like Compass, Sprite Cow, or SpritePad
- Organize icons logically in your sprite sheet
- Document your sprite positions
- Consider maintaining separate sprite sheets for different sections of your site
- Use SVG sprites for scalable icons when appropriate
Conclusion
CSS Sprites demonstrate how clever engineering can turn a simple concept into a powerful optimization technique. While newer technologies have emerged, the fundamental benefits of reducing HTTP requests and improving caching efficiency make CSS Sprites a valuable tool in any web developer's arsenal.
By understanding and implementing this technique, you can create faster, more efficient websites that provide a better user experience. Whether you're building the next Facebook or optimizing your personal blog, CSS Sprites are worth considering as part of your performance optimization strategy.
Remember, in web development, small optimizations can lead to significant improvements when scaled across millions of users. CSS Sprites are a perfect example of this principle in action.